Connect with us!
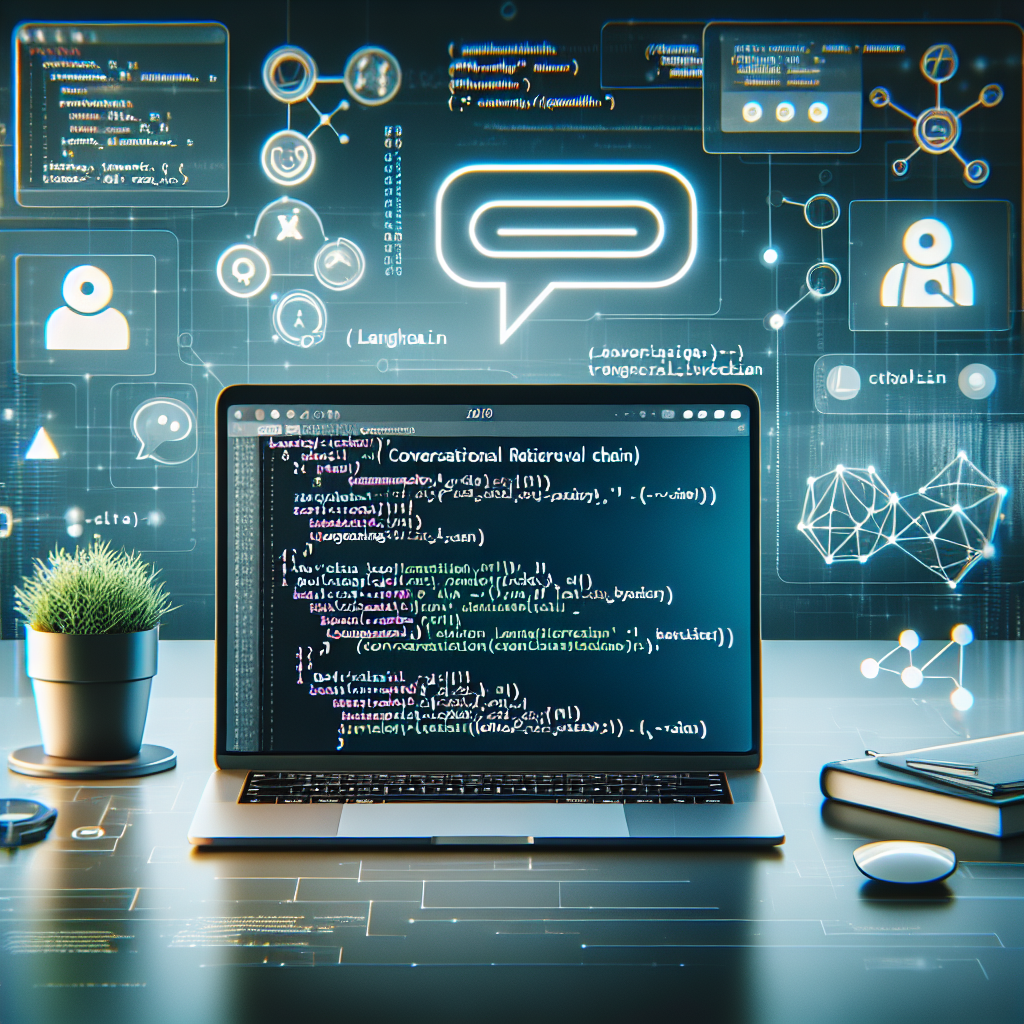
Enhance Langchain ConversationalRetrievalChain with Prompt and Chat History
In this tutorial, we’ll walk you through enhancing Langchain’s ConversationalRetrievalChain with prompt customization and chat history management. Let’s dive into this step-by-step guide and make your conversational agents even more powerful.
Table of Contents
- Introduction
- Prerequisites
- Setting Up Langchain
- Customizing the Prompt
- Managing Chat History
- Putting It All Together
- Conclusion
Introduction
Langchain’s ConversationalRetrievalChain is an advanced tool for building conversational AI systems that can retrieve and respond to user queries. Enhancing these capabilities with prompt customization and chat history can significantly improve the quality of interactions. By doing so, you’ll ensure your conversational agent not only retrieves the relevant information but also maintains context throughout the conversation.
Prerequisites
Before we start, ensure you have the following:
- Basic knowledge of Python
- Familiarity with Langchain library
- Installed versions of Python 3.x
- PIP for managing Python packages
Setting Up Langchain
First, you need to set up the Langchain library. If you haven’t already, you can install it via pip:
pip install langchain
Initializing the ConversationalRetrievalChain
Let’s initialize a simple ConversationalRetrievalChain:
from langchain.chains import ConversationalRetrievalChain
chain = ConversationalRetrievalChain()
Customizing the Prompt
Customizing the prompt allows you to set the initial conditions and context of the conversation. This helps in guiding the AI to generate more contextually accurate responses.
Example of Prompt Customization
Here’s a basic example:
from langchain.prompts import PromptTemplate
prompt = PromptTemplate(
input_variables=["context", "question"],
template="You are a helpful assistant. Given the context: {context}, answer the question: {question}"
)
chain.set_prompt(prompt)
Managing Chat History
Maintaining chat history is crucial for delivering coherent and context-aware responses. Langchain provides utilities to handle chat history efficiently.
Implementing Chat History
Here’s how you can manage chat history:
from langchain.memory import ChatMemory
memory = ChatMemory()
# Adding to memory
memory.add_message(user="user", text="What’s the weather like today?")
memory.add_message(user="assistant", text="The weather is sunny with a few clouds.")
# Retrieving from memory
history = memory.get_memory()
Putting It All Together
Now, let’s combine prompt customization and chat history into the ConversationalRetrievalChain:
from langchain.chains import ConversationalRetrievalChain
from langchain.prompts import PromptTemplate
from langchain.memory import ChatMemory
# Initialize chat memory
memory = ChatMemory()
# Add initial messages
memory.add_message(user="user", text="What's the capital of France?")
memory.add_message(user="assistant", text="The capital of France is Paris.")
# Customizing the prompt
prompt = PromptTemplate(
input_variables=["context", "question"],
template="You are a helpful assistant. Given the context: {context}, answer the question: {question}"
)
# Initialize the chain with prompt and memory
chain = ConversationalRetrievalChain(prompt=prompt, memory=memory)
# Example usage
response = chain.process_question("Can you tell me more about Paris?", context="France is a country in Europe.")
Conclusion
By customizing the prompt and managing chat history in your Langchain ConversationalRetrievalChain, you can create a more robust and contextually aware conversational agent. This approach ensures that your AI can handle conversations fluidly and effectively, improving the overall user experience.
Happy coding!