Connect with us!
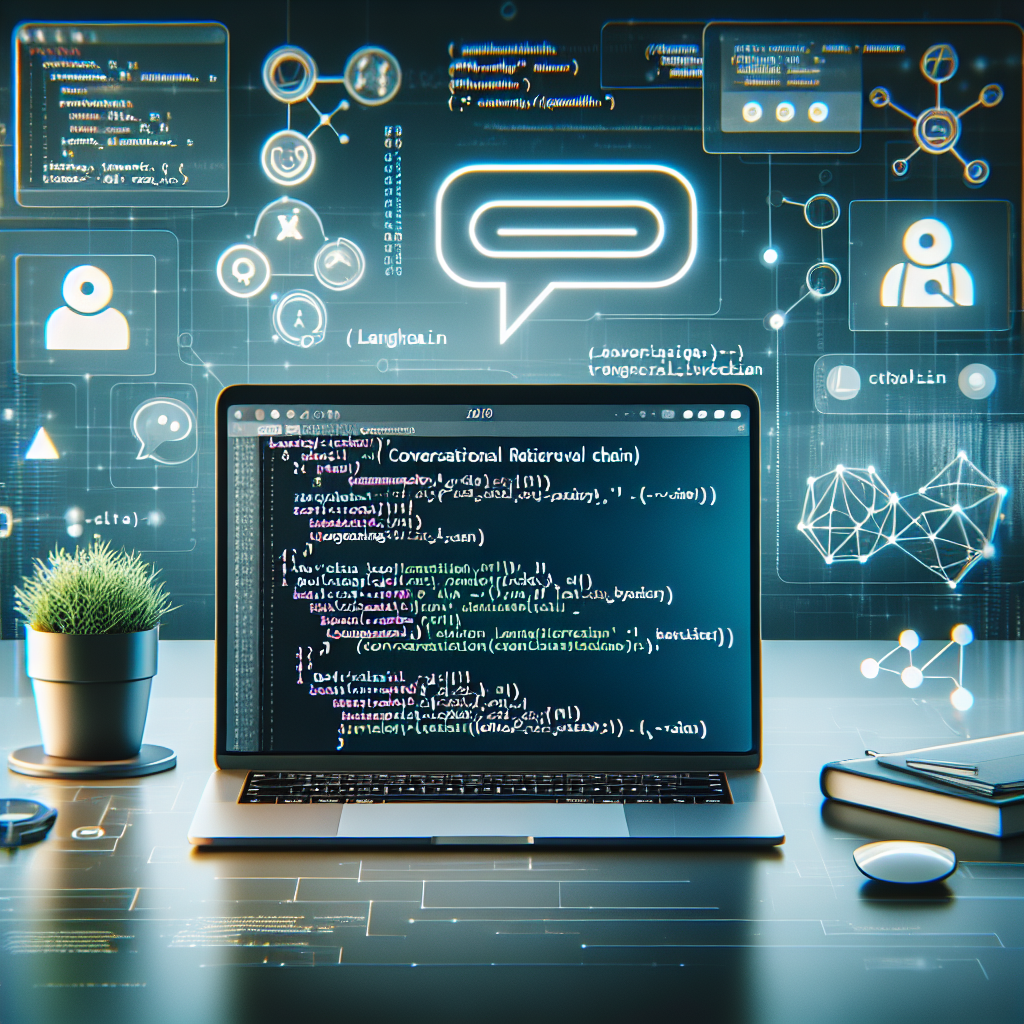
Ensuring Complete Responses from OpenAI API: A Step-by-Step Guide
Working with the OpenAI API can be incredibly rewarding, but sometimes you might find yourself struggling to get complete responses to your queries. This tutorial will guide you through the steps needed to ensure that you get the most comprehensive and accurate results from your API interactions.
Why Ensure Complete Responses?
OpenAI’s powerful models can generate detailed and contextually rich responses. However, without proper setup, you might run into issues such as truncated text or incomplete answers. Ensuring complete responses enhances the utility and reliability of your application.
Step-by-Step Guide
1. Choose the Appropriate Model
Different OpenAI models excel at different types of tasks. For instance:
- GPT-3 Davinci: Best for complex and nuanced responses.
- GPT-3 Curie: Great for text completion and generating conversational responses.
- GPT-3 Babbage: Suitable for straightforward tasks and less complex queries.
- GPT-3 Ada: Fastest and cost-effective for simple tasks.
Select a model that aligns with your specific use case. For detailed and thorough responses, Davinci is generally the best choice.
2. Set the Right Parameters
**Parameters** play a crucial role in the completeness and quality of responses. Here are the key parameters to focus on:
- Max Tokens: Ensure that you set a sufficiently high max token limit. This controls the maximum length of the output:
{
"max_tokens": 1500
} - Temperature: Controls the randomness of the output. A lower temperature (e.g., 0.3) produces more deterministic responses, which might be useful for consistency:
{
"temperature": 0.3
} - Top_p: An alternative to temperature sampling called nucleus sampling. A lower value like 0.7 ensures that the most probable tokens are considered, which can lead to more complete answers:
{
"top_p": 0.7
} - Frequency_penalty and Presence_penalty: Adjust these to penalize repetitive responses:
{
"frequency_penalty": 0.5,
"presence_penalty": 0.5
}
3. Crafting Effective Prompts
**Prompts** are the backbone of getting useful responses from the OpenAI API. Here are tips for crafting effective prompts:
- **Be Specific:** Instead of asking a general question, be as specific as possible.
- **Provide Context:** Include background information that the model can use to generate a relevant response.
- **Use Examples:** If applicable, provide examples to guide the model towards the desired format or structure.
**Example Prompt:**
"Write a detailed summary of the events leading up to the signing of the Declaration of Independence, including key figures and their contributions."
4. Handling Incomplete Responses
Sometimes, despite your best efforts, responses may still be incomplete. To handle this:
- **Check Response Length:** Programmatically check if the response is cut off, indicating the max token limit was reached.
- **Follow-up Queries:** Submit a follow-up prompt to ask for additional details or continuation.
- **Use Stop Sequences:** Implement stop sequences to make sure the response ends logically:
{
"stop": ["\n", "End of response"]
}
5. Error Handling and Retries
Robust error handling is crucial for production applications. Implement strategies to:
- **Retry Failed Requests:** Automatically retry API calls in case of network or server issues.
- **Log Errors:** Maintain logs of all errors for troubleshooting and improvement.
Example of a retry mechanism in Python:
import time
import openai
def call_openai_api(prompt, retries=3):
for attempt in range(retries):
try:
response = openai.Completion.create(
engine="davinci",
prompt=prompt,
max_tokens=1500
)
return response.choices[0].text
except Exception as e:
print(f"Attempt {attempt + 1} failed: {e}")
time.sleep(2)
return "API call failed after retries."
response = call_openai_api("What were the causes of the American Civil War?")
print(response)
Conclusion
Ensuring complete responses from the OpenAI API involves selecting the right model, setting appropriate parameters, crafting effective prompts, and implementing robust error handling. By following this guide, you can optimize your API interactions for detailed and comprehensive outputs, making your application more reliable and user-friendly.
Feel free to share your experiences or ask questions in the comments below. Happy coding!