Connect with us!
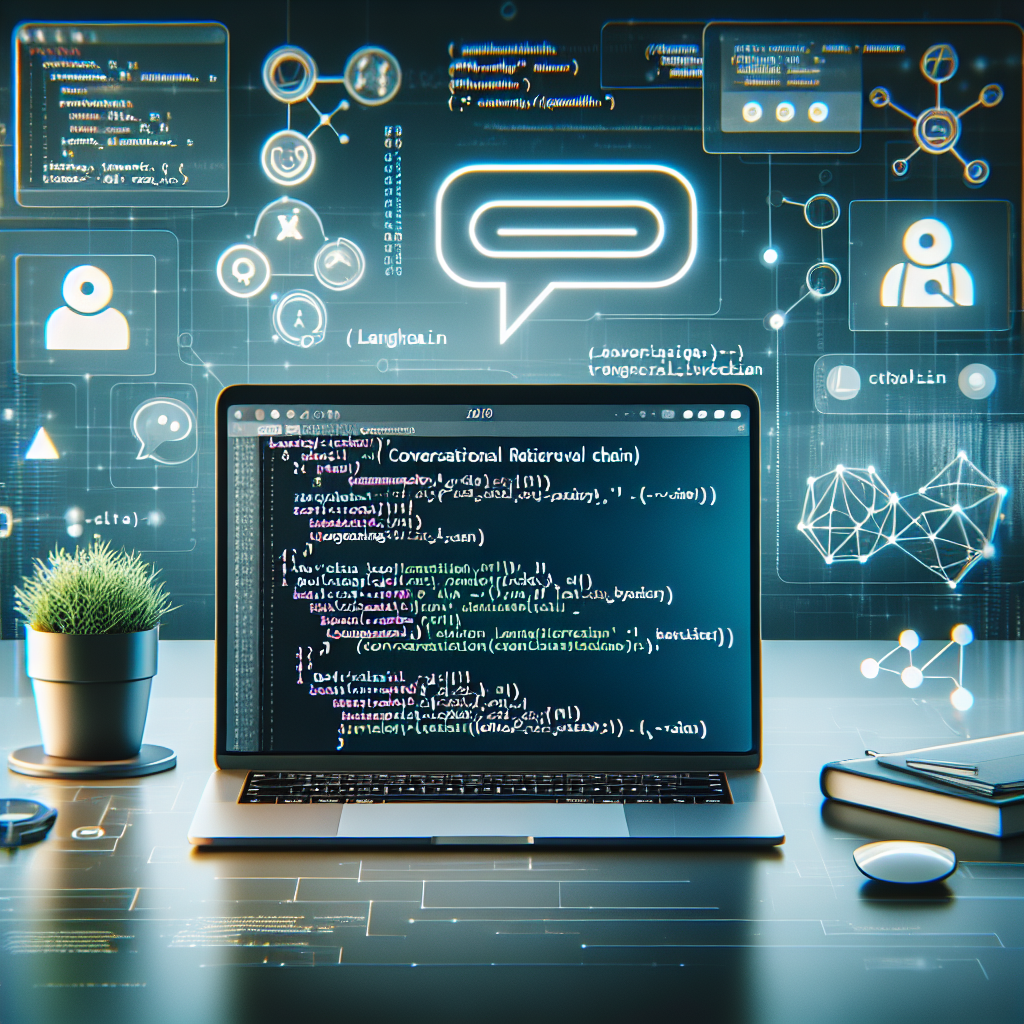
OpenAI API ChatCompletion Error: Solutions for Version 1.0.0 and Above
The OpenAI API has become a powerful tool for developers looking to implement advanced language models into their projects. However, with its complexity, issues and errors are common, especially with the introduction of new versions. This article discusses common ChatCompletion errors encountered in Version 1.0.0 and above, providing practical solutions to help you overcome these roadblocks.
Table of Contents
- Common Errors and Their Causes
- Error Handling Best Practices
- Step-by-Step Troubleshooting Guide
- Advanced Debugging Techniques
- Conclusion
Common Errors and Their Causes
Understanding the root cause of an error is half the battle. Below are some common errors you may encounter when using the OpenAI API ChatCompletion feature:
1. API Key Error
Description: “Invalid API key provided.”
Cause: The API key used is either incorrect or expired.
Solution: Double-check the API key you are using. Ensure it is still active and correctly input.
2. Rate Limit Error
Description: “Rate limit reached.”
Cause: You have exceeded the number of allowed requests within a specified timeframe.
Solution: Implement rate limiting in your code to manage requests. You can also consider upgrading your plan for higher rate limits.
3. Input Token Length Error
Description: “Input exceeds maximum token length.”
Cause: The input text is too long and surpasses the token limit imposed by OpenAI.
Solution: Pre-process and shorten your input text. Alternatively, split your text into smaller chunks and make multiple API calls.
4. Model Not Available
Description: “The specified model is not available.”
Cause: The model you are attempting to use is either deprecated or not accessible in your plan.
Solution: Check the OpenAI documentation for available models and update your request accordingly.
Error Handling Best Practices
To minimize disruptions and maintain a smooth user experience, follow these best practices:
Implement Retries with Backoff
- Steps:
- Define a maximum number of retry attempts.
- Use exponential backoff strategy for retries to avoid spamming the server.
Validate Inputs
- Steps:
- Validate input length and content before making an API call.
- Ensure your inputs adhere to the requirements specified by OpenAI.
Monitor Usage
- Steps:
- Regularly monitor your API usage and rate limits.
- Utilize logging to keep track of request patterns and identify potential issues early.
Step-by-Step Troubleshooting Guide
When an error occurs, follow these steps to troubleshoot:
Step 1: Check API Key and Configuration
Ensure that your API key and configuration are correctly set.
import openai
openai.api_key = 'your-api-key'
Step 2: Review Documentation
Consult the OpenAI API documentation for any updates or changes that might affect your implementation.
Step 3: Test with Basic Inputs
Use a simple, basic input to determine if the issue lies with your data.
response = openai.ChatCompletion.create(
model="text-davinci-002",
messages=[{"role": "user", "content": "Hello, how are you?"}]
)
Step 4: Log and Debug
Implement detailed logging to capture error messages and responses for further analysis.
import logging
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger(__name__)
try:
response = openai.ChatCompletion.create(
model="text-davinci-002",
messages=[{"role": "user", "content": "Hello, how are you?"}]
)
logger.debug(response)
except Exception as e:
logger.error(f"Error occurred: {e}")
Advanced Debugging Techniques
For more challenging issues, consider the following advanced techniques:
Use the OpenAI API Tools
OpenAI offers several tools to help developers test and debug API calls directly from their platform.
Monitor Network Traffic
Use tools like Wireshark or Postman to examine network traffic and verify API request and response details.
Community and Support
Engage with community forums or contact OpenAI support for insights and assistance on persistent issues.
Conclusion
Encountering errors while using OpenAI’s ChatCompletion feature can be frustrating, but understanding common issues and having a plan for troubleshooting can greatly ease the process. By following the guidance provided in this article, you’ll be well-equipped to handle errors in Version 1.0.0 and beyond. Remember to maintain good practices, such as input validation and logging, to preempt potential issues and ensure a smoother user experience.
For further reading, continue to explore OpenAI’s official documentation and join developer communities to stay updated on best practices and new developments.