Connect with us!
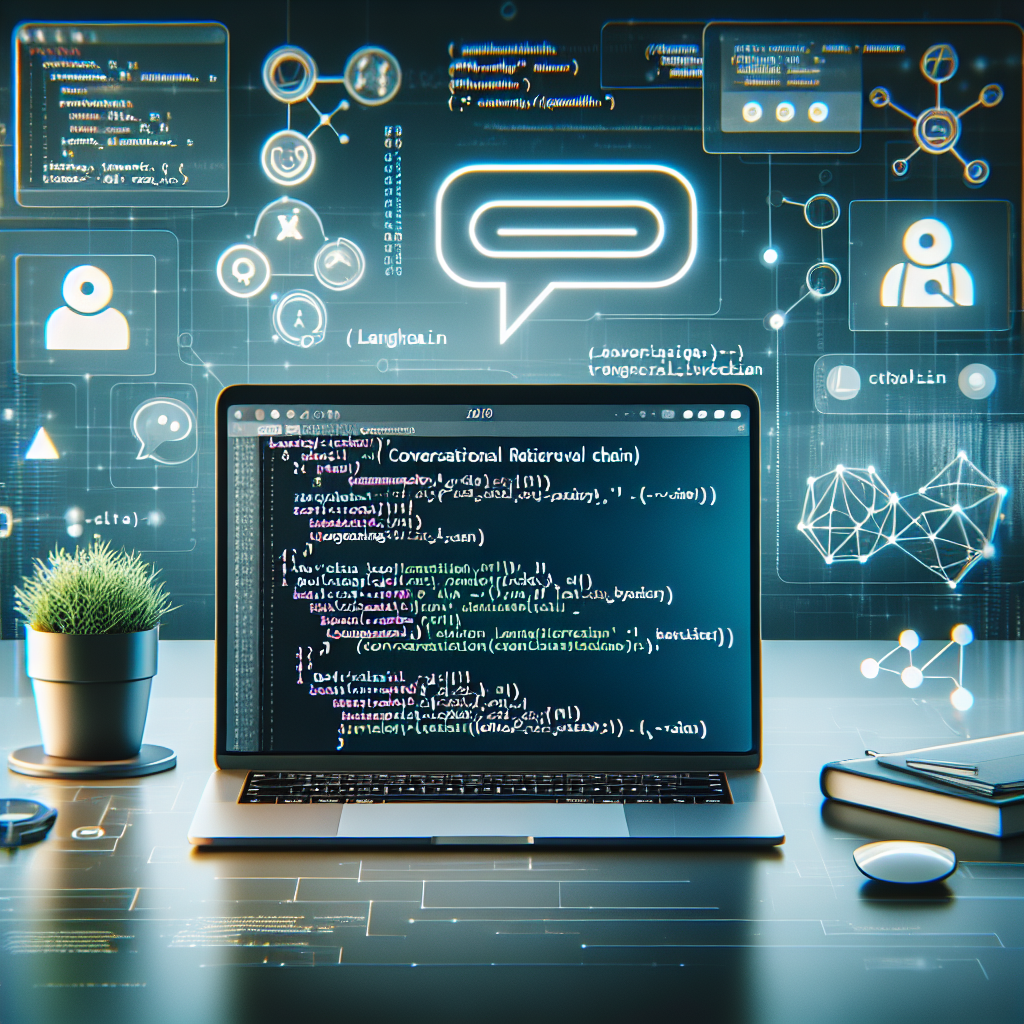
Understanding the Loss Function in Hugging Face’s Transformers Trainer
Table of Contents
- Introduction
- What is a Loss Function?
- Types of Loss Functions
- Hugging Face’s Transformers and Trainer
- Implementing the Loss Function in Hugging Face’s Transformers Trainer
- Conclusion
Introduction
In the captivating world of machine learning, understanding the mechanics behind various components is crucial for developing effective models. One such essential component is the loss function. This tutorial aims to provide a detailed walkthrough of the loss function within Hugging Face’s Transformers Trainer framework.
What is a Loss Function?
A loss function is a measure of how well or poorly a machine learning model is performing. It evaluates the difference between the predicted output and the actual output. Depending on the model’s performance, the loss function generates a numerical value which the training algorithm then uses to update model parameters to minimize this loss.
Importance of Loss Functions
- Model Optimization: Guide the optimization process by providing a gradient to drive parameter updates.
- Performance Metric: Serve as a metric for assessing model performance during and after training.
Types of Loss Functions
Different machine learning tasks utilize various types of loss functions. Here are a few commonly used loss functions:
Mean Squared Error (MSE)
MSE is used for regression tasks. It measures the average squared difference between the actual and predicted values.
Cross-Entropy Loss
Cross-entropy loss, also known as log loss, is widely used for classification tasks. It evaluates the performance of a classification model by measuring the divergence between predicted probabilities and true labels.
Huber Loss
Huber loss is a combination of MSE and Mean Absolute Error (MAE) and is less sensitive to outliers than MSE.
Hugging Face’s Transformers and Trainer
Hugging Face has revolutionized the Natural Language Processing (NLP) landscape with its Transformers library. It provides state-of-the-art pre-trained models for tasks like text classification, translation, named entity recognition, and more.
Transformers Library
The Transformers library simplifies the usage of transformer models. Pre-trained models can be fine-tuned for specific tasks without extensive configuration.
The Trainer Class
The Trainer class in Hugging Face’s library provides an easy-to-use training interface. It handles the training loop, evaluation, and prediction. Key features include:
- Automatic logging of performance metrics.
- Integrated gradient accumulation for handling large batches.
- Support for various optimizers and learning rate schedulers.
Implementing the Loss Function in Hugging Face’s Transformers Trainer
To implement a custom loss function in Hugging Face’s Transformers Trainer, follow these steps:
Step 1: Setup and Installation
Ensure you have the Transformers library installed. You can install it using pip:
pip install transformers
Step 2: Load Pre-trained Model and Tokenizer
Load a pre-trained model and corresponding tokenizer:
from transformers import AutoModelForSequenceClassification, AutoTokenizer
model_name = "bert-base-uncased"
model = AutoModelForSequenceClassification.from_pretrained(model_name)
tokenizer = AutoTokenizer.from_pretrained(model_name)
Step 3: Custom Loss Function
Define a custom loss function. For instance, if you want to use mean squared error:
import torch.nn as nn
class CustomMSELoss(nn.Module):
def __init__(self):
super(CustomMSELoss, self).__init__()
self.mse_loss = nn.MSELoss()
def forward(self, outputs, labels):
return self.mse_loss(outputs.logits, labels)
Step 4: Integrate Custom Loss Function
Integrate the custom loss function into the Trainer class:
from transformers import Trainer, TrainingArguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
warmup_steps=500,
weight_decay=0.01,
logging_dir='./logs',
logging_steps=10,
)
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=eval_dataset,
compute_metrics=compute_metrics,
loss_fn=CustomMSELoss()
)
Step 5: Train and Evaluate the Model
Train the model and evaluate its performance:
trainer.train()
eval_results = trainer.evaluate()
print(eval_results)
Conclusion
Understanding and effectively leveraging the loss function is vital for training robust and accurate machine learning models. Hugging Face’s Transformers Trainer simplifies this process by providing a streamlined training interface. By following this tutorial, you are now equipped to implement and customize loss functions to optimize your models’ performance effectively.
Happy training!